API Integration of MobileMatch
Overview
This guide will show you how to use the AXN Verify API to verify an individual by MobileMatch.
Prerequisites
- Deploy the "MobileMatch" Workflow by following the directions here.
- Obtain the access keys for your new workflow by following the directions here
- Download and use the MobileMatch Postman Project to try it out
What you'll do:
- Collect the Full Name, Home Address and Personal Mobile Phone Number of the end user.
- Ensure that the full name and/or address are associated with the phone number (Step 2).
- Ensure that the end user has possession of the device (Steps 3 and 4).
1. Get Access Token
(POST) https://api.preprod.iddataweb.com/v1/token
API Reference: https://docs.iddataweb.com/reference/auth
This access token is used to authenticate your requests. Place this token in the header of each subsequent request. See the postman project above for examples.
Locate your workflow's access keys
Then, to request an access token:
var axios = require('axios')
var user = 'Your Service API Key';
var password = 'Your Service Shared Secret';
var base64encodedData = Buffer.from(user + ':' + password).toString('base64');
var request = async () => {
var response = await axios({
url: 'https://api.preprod.iddataweb.com/v1/token',
method: 'post',
params: {
grant_type: 'client_credentials'
},
headers: {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
Authorization: 'Basic ' + base64encodedData
}
})
console.log(response.data)
}
request();
Response
{
"errorCode": "",
"errorDescription": "",
"access_token": "TjZK7NSjw45AxGK9UhOE9uJDaZT23gKYuUC-9GMTAgLU", // your access token
"expires_in": "1800",
"token_type": "Bearer"
}
1.1. Country Selection
(POST) https://api.preprod.iddataweb.com/v1/slverify
API Reference: https://docs.iddataweb.com/reference/slverify
Once you've received an access token, you'll need to check if the user is located in a country supported by our services.
Using the same API Key as before, prompt the user to select a country, and if the country is supported, your response will contain the API Key required to take the next step in MobileMatch verification.
Request
var data = {
"apikey": "Your API Key",
"credential": "e.g. : [email protected]",
"appID":"Your App Name, (e.g. 'Employee Onboarding App')",
"userAttributes": [
{
"attributeType": "Country",
"values": {
"country": "US" // accepted values: (country codes) e.g. "US", "MX", "AU" ....
}
}
]
}
var axios = require('axios')
var token = 'YOUR_BEARER_TOKEN' // -- obtained from step 1 --
var request = async () => {
var response = await axios({
url: 'https://api.preprod.iddataweb.com/v1/slverify',
method: 'post',
data: data,
headers: {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
Authorization: 'Bearer ' + token,
}
})
console.log(response.data)
}
request();
Response
{
"errorCode": "",
"errorDescription": "",
"transaction_id": "xxxxx-xxxx-xxxx-xxxx-xxxxx",
"userAttributes": [
{
"attributeType": "Country",
"dateCreated": "11/08/2022 17:26:36",
"values": {
"country": "US"
}
}
],
"acquiredAttributes": null,
"userAssertionList": [
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"dateAsserted": "11/08/2022 17:26:37",
"assertions": {
"blacklist.device": "unverified",
"blacklist.ofacIP": "unverified",
"test.gte3Credential1d": "unverified",
"detect.browserAnomaly": "unverified",
"test.gte5Credential1d": "unverified",
"test.lte3ProxyToday": "unverified",
"test.trustedDevice6mo": "unverified",
"test.trustedDevice": "unverified",
"detect.possibleVPNOrTunnel": "unverified",
"test.lte3CredentialsDevice7d": "unverified",
"test.gte5Device1d": "unverified",
"link.timeZone_TrueGeo": "unverified",
"test.gte5CredentialDevice1d": "unverified",
"test.credentialLTE500mi1hr": "unverified",
"detect.vpn": "unverified",
"test.exactIDAgeGTE7d": "unverified",
"test.trueIPLTE500miInputIP": "unverified",
"detect.proxyAnonymous": "unverified",
"test.apSessionIDNotReplay": "unverified",
"detect.jailbreak": "unverified",
"link.proxyOrg_TrueOrg": "unverified",
"test.expectedLanguage": "unverified",
"blacklist.ip": "unverified",
"detect.malware": "unverified",
"detect.torExitNode90d": "unverified",
"test.gte10Credential1d": "unverified",
"detect.mobileTethering": "unverified",
"test.trustedDevice28days": "unverified",
"test.gte20Credential1d": "unverified",
"detect.aggregator": "unverified",
"test.smartIDAgeGTE7d": "unverified",
"detect.proxyOpenTransparent": "unverified",
"detect.unusualActivity": "unverified",
"detect.proxyHidden": "unverified",
"link.proxyISP_TrueISP": "unverified",
"detect.tor": "unverified",
"detect.torNode": "unverified",
"detect.knownVPNISP": "unverified",
"link.proxyGeo_TrueGeo": "unverified",
"test.gte2Credential1d": "unverified"
}
}
],
"mbun": "xxxxxx-xxxx-xxxx-xxxx-xxxxxx",
"forwardApiKey": "324e1d4975f40c5", // Your Next API Key
"policyObligation": true,
"policyDecision": "obligation"
}
NOTE:
The output above includes additional information from our Device Profiling service.
Device Profiling is the process of screening the Device and User Activity of a new transaction (user session).
If a transaction is found to be high risk (fraud), you'll be alerted of any fraudulent activity found, and the transaction will be prevented from proceeding further. Device Profiling requires an embedded script to run. Without it, assertions will be marked "unverified".
2. Collect Personal Information (PII)
(POST) https://api.preprod.iddataweb.com/v1/slverify
API Reference: https://docs.iddataweb.com/v1.0/reference/slverify
/slverify
collects the individual's Personal Information and Phone Number, and returns a policyDecision indicating how accurately this information matches.
-
If the individual's Full Name and Phone Number match active phone records,
/slverify
will return a policyDecision of obligation, and also allow them to receive an OTP (via SMS or voice). -
If the individual's Full Name and Phone Number do not match,
/slverify
will return a policyDecision of deny, and the workflow will not continue.
Each step has a designated API Key.
Each key is designed to return the API key required for the next step. This key is called your forwardApiKey.
In the response body of your last request, you'll find your next forwardApiKey.
Request
var axios = require('axios')
var token = 'YOUR_BEARER_TOKEN' // -- obtained from step 1 --
var data = {
"apikey": "YOUR_MOBILEMATCH_PII_API_KEY", // -- obtained from step 1.1 --
"credential": "USERNAME",
"appID":"YOUR_APPLICATION_NAME",
"asi": "xxxxx-xxxx-xxxx-xxxxx", // -- obtained from step 1.1 --
"userAttributes": [
{
"attributeType": "FullName",
"values": {
"fname": "John",
"mname": "",
"lname": "Smith"
}
},
{
"attributeType": "InternationalAddress",
"values": {
"country": "US",
"administrative_area_level_1": "VA",
"locality": "Vienna",
"postal_code": "23225",
"route": "Liberty St",
"street_number": "123"
}
},
{
"attributeType": "InternationalTelephone",
"values": {
"dialCode": "1",
"telephone": "1234567890"
}
}
]
}
var request = async () => {
var response = await axios({
url: 'https://api.preprod.iddataweb.com/v1/slverify',
method: 'post',
data: data,
headers: {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
Authorization: 'Bearer ' + token
}
})
console.log(response.data)
}
request();
Response
{
"errorCode": "",
"errorDescription": "",
"transaction_id": "xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxx",
"userAttributes": [
{
"attributeType": "FullName",
"dateCreated": "10/27/2022 13:50:14",
"values": {
"fname": "John",
"lname": "Smith",
"mname": "",
"suffix": null
}
},
{
"attributeType": "InternationalAddress",
"dateCreated": "10/27/2022 13:50:14",
"values": {
"country": "US",
"sublocality": null,
"locality": "Vienna",
"subpremise": null,
"sublocality_level_2": null,
"route": "Liberty St",
"administrative_area_level_2": null,
"premise": null,
"sublocality_level_5": null,
"administrative_area_level_3": null,
"sublocality_level_4": null,
"sublocality_level_3": null,
"administrative_area_level_1": "VA",
"street_number": "123",
"neighborhood": null,
"postal_code": "23225"
}
},
{
"attributeType": "InternationalTelephone",
"dateCreated": "10/27/2022 13:50:14",
"values": {
"dialCode": "1",
"telephone": "1234567890"
}
}
],
"acquiredAttributes": [
{
"provider": "Experian",
"serviceOffering": "Experian Precise ID",
"attributeType": "ExperianScore",
"dateCreated": "10/27/2022 13:50:15",
"values": {
"experianScore": "488"
}
},
{
"provider": "Experian",
"serviceOffering": "Experian Precise ID",
"attributeType": "MostRecentAddress",
"dateCreated": "10/27/2022 13:50:15",
"values": {
"country": null,
"address": null,
"route": null,
"administrative_area_level_1": null,
"street_number": null,
"locality": null,
"postal_code": null,
"subpremise": null
}
}
],
"userAssertionList": [
{
"provider": "Experian",
"serviceOffering": "Experian Precise ID",
"dateAsserted": "10/27/2022 13:50:15",
"assertions": {
"test.fraudulentActivityAddress": "pass",
"link.fullName_address": "fail",
"link.lastName_address": "fail",
"test.noAddressConflicts": "pass",
"test.address90DaysOld": "pass",
"test.fileOneAddressMatch": "pass",
"test.addressIsResidential": "fail",
"link.lastName_phone": "fail",
"link.phone_address": "fail",
"link.fullName_phone": "fail"
}
},
{
"provider": "Boku",
"serviceOffering": "Boku - International Mobile Carrier Reverse Lookup",
"dateAsserted": "10/27/2022 13:50:15",
"assertions": {
"link.phone_zip": "unverified",
"test.lastPortGT14days": "unverified",
"test.phone_landline_mobile_personal": "unverified",
"test.phoneActive": "unverified",
"test.lastPortGT30days": "unverified",
"link.lastName_phone": "unverified",
"link.phone_address": "unverified",
"link.phone_state": "unverified",
"link.fullName_phone": "unverified"
}
}
],
"mbun": "xxxxxx-xxxx-xxxx-xxxx-xxxxxx",
"forwardApiKey": "YOUR_FORWARD_API_KEY", // If you've successfully verified PII, this will be your OTP API key. If not, the API key to verify PII will be returned so that you can try again.
"policyObligation": true,
"policyDecision": "obligation"
}
3. A) Send FastTap Link
(GET) https://api.preprod.iddataweb.com/v1/doccapture/sendlink
API Reference: https://api.preprod.iddataweb.com/v1/doccapture/sendlink
You can request a FastTap MFA link by specifying the Dial code and Phone number to send to, and authenticating the request using the Bearer token obtained via. step 1.
Request
var axios = require("axios");
var token = "YOUR_BEARER_TOKEN"; // -- obtained from step 1 --
var params = {
dialCode: "1",
telephone: "1234567890",
apikey: "YOUR_FASTTAP_MFA_API_KEY",
credential: "USERNAME",
appID: "YOUR_APPLICATION_NAME",
};
var request = async () => {
var response = await axios({
url: "https://api.preprod.iddataweb.com/v1/doccapture/sendlink",
method: 'get',
params: params,
headers: {
"Content-Type": "application/json",
"Cache-Control": "no-cache",
Authorization: "Bearer " + token,
},
});
console.log(response.data);
};
request();
Response
{
responseCode: '200',
errorDescription: null,
asi: 'xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxx',
status: 'SUCCESS'
}
3. B) Send FastTap Link w/ Custom Text
(POST) https://api.preprod.iddataweb.com/v1/doccapture/sendlink
API Reference: https://api.preprod.iddataweb.com/v1/doccapture/sendlink
Optionally, you can also send custom text along with your FastTap link to change the content of the page users visit.
Request
var axios = require("axios");
var token = "YOUR_BEARER_TOKEN"; // -- obtained from step 1 --
var data = {
dialCode: "1",
telephone: "1234567890",
apikey: "YOUR_FASTTAP_MFA_API_KEY",
credential: "USERNAME",
appID: "YOUR_APPLICATION_NAME",
explanationHTML: "Hello, in order to to complete your transfer of <b>$___</b> from account #1234 to <b>Account Name</b>, please click the Continue button below"
};
var request = async () => {
var response = await axios({
url: "https://api.preprod.iddataweb.com/v1/doccapture/sendlink",
method: 'post',
data: data,
headers: {
"Content-Type": "application/json",
"Cache-Control": "no-cache",
Authorization: "Bearer " + token,
},
});
console.log(response.data);
};
request();
Response
{
responseCode: '200',
errorDescription: null,
asi: 'xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxx',
status: 'SUCCESS'
}
Output
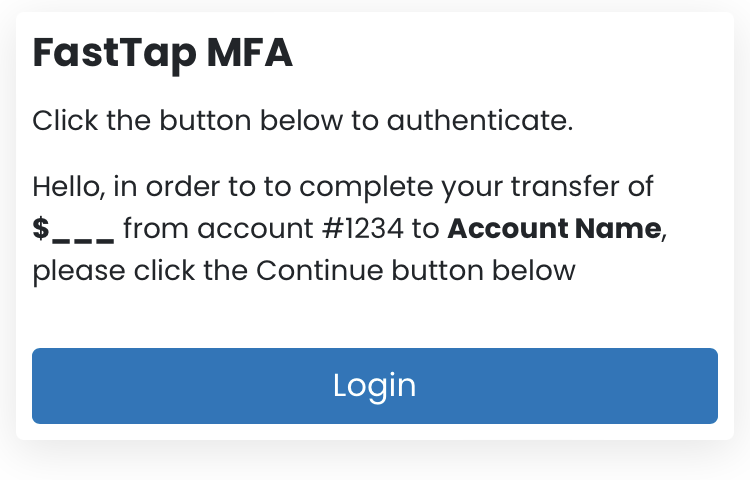
4. Get FastTap Status
(Get) https://api.preprod.iddataweb.com/v1/doccapture/results
API Reference: https://api.preprod.iddataweb.com/v1/doccapture/results
Confirm the user's completion of FastTap.
Periodically, resend /doccapture/results to confirm the status of the FastTap link. We advise this be done every 3-5 seconds.
Request
var axios = require("axios");
var token = "YOUR_BEARER_TOKEN"; // -- obtained from step 1 --
var params = {
asi: "YOUR_SESSION_ID" // -- obtained from step 3 --
};
var request = async () => {
var response = await axios({
url: "https://api.preprod.iddataweb.com/v1/doccapture/results",
method: 'get',
params: params,
headers: {
"Content-Type": "application/json",
"Cache-Control": "no-cache",
Authorization: "Bearer " + token,
},
});
console.log(response.data);
};
request();
Response
{
"responseCode": null,
"errorDescription": "Status is not yet complete.",
asi: 'xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxx',
"status": "pending",
"urls": {}
}
// OR
{
"responseCode": "200",
"errorDescription": "",
asi: 'xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxx',
"status": "success",
"urls": {}
}
5. Verify FastTap
(POST) https://api.preprod.iddataweb.com/v1/slverify
API Reference: https://api.preprod.iddataweb.com/v1/slverify
Lastly, confirm the user's session (asi) and Phone number used to complete verification.
Request
var axios = require("axios");
var token = "YOUR_BEARER_TOKEN"; // -- obtained from step 1 --
var data = {
"asi": "xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxx", // -- obtained from step 1.1 --
"apikey": "YOUR_FASTTAP_MFA_API_KEY", // -- obtained from step 3 --
"credential": "USERNAME",
"appID":"YOUR_APPLICATION_NAME",
"userAttributes": [
{
"attributeType": "InternationalTelephone",
"values": {
"dialCode": "your-dial-code",
"telephone": "your-phone-number"
}
},
{
"attributeType": "PINDeliveryPreference",
"values": {
"pindeliverypreference": "SMS" // accepted values: "voice", "SMS"
}
}
]
}
var request = async () => {
var response = await axios({
url: "https://api.preprod.iddataweb.com/v1/slverify",
method: 'post',
data: data,
headers: {
"Content-Type": "application/json",
"Cache-Control": "no-cache",
Authorization: "Bearer " + token,
},
});
console.log(response.data);
};
request();
Response
{
"errorCode": "",
"errorDescription": "",
"transaction_id": "xxxx-xx-xxxx-xx-xxxxx",
"userAttributes": [
{
"attributeType": "InternationalTelephone",
"dateCreated": "07/07/2023 18:23:51",
"values": {
"dialCode": "",
"telephone": ""
}
}
],
"acquiredAttributes": [
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "FuzzyDeviceID",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"fuzzyDeviceID": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TMXScore",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"tmxScore": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "DigitalIDConfidence",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"digitalIDConfidence": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIPGeoCountry",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIPGeoCountry": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "BiometricFraudScore",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"biometricFraudScore": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIP",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIP": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "OperatingSystemAnomaly",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"operatingSystemAnomaly": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "ScreenResolution",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"screenResolution": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "BiometricBotScore",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"biometricBotScore": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "SmartIDBrowserstringPersonaAgeMonths",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"smartIDBrowserStringPersonaAge": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "HTMLLocationAccuracy",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"htmlLocationAccuracy": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIPConnectionType",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIPConnectionType": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "DigitalID",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"digitalID": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "BiometricAnomalyScore",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"biometricAnomalyScore": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "HoneypotFingerprintMatch",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"honeypotFingerprintMatch": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "ExactIDIPPersonaAgeMonths",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"exactIDIPPersonaAge": "0"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIPCity",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIPCity": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIPLineSpeed",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIPLineSpeed": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "OperatingSystem",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"operatingSystem": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "SocialEngineeringScore",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"socialEngineeringScore": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "HTMLLocationLongitude",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"htmlLocationLongitude": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "BiometricAssessmentScore",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"biometricAssessmentScore": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "BrowserSpoofReason",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"browserSpoofReason": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "HTMLLocationLatitude",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"htmlLocationLatitude": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIPLongitude",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIPLongitude": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "OperatingSystemVersion",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"operatingSystemVersion": ""
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "BiometricReasonCode",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"biometricReasonCode": "Not Found"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "NEATPersonaAgeMonths",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"NEATPersonaAge": "0"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "FuzzyDeviceIDConfidence",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"fuzzyDeviceIDConfidence": "100"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "Platform",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"platform": "browser_mobile"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"attributeType": "TrueIPLatitude",
"dateCreated": "07/07/2023 18:23:53",
"values": {
"trueIPLatitude": ""
}
}
],
"userAssertionList": [
{
"provider": "Telesign",
"serviceOffering": "Telesign Phone Contact API",
"dateAsserted": "07/07/2023 18:23:52",
"assertions": {
"test.phone_landline_mobile_personal": "unverified",
"link.lastName_phone": "unverified",
"link.fullName_phone": "unverified"
}
},
{
"provider": "Threatmetrix",
"serviceOffering": "Threatmetrix Session Query Low Location Accuracy",
"dateAsserted": "07/07/2023 18:23:53",
"assertions": {
"blacklist.device": "pass",
"blacklist.ofacIP": "pass",
"test.gte3Credential1d": "pass",
"detect.browserAnomaly": "pass",
"test.gte5Credential1d": "pass",
"test.lte3ProxyToday": "pass",
"test.trustedDevice6mo": "fail",
"test.trustedDevice": "fail",
"test.lte3CredentialsDevice7d": "pass",
"detect.possibleVPNOrTunnel": "pass",
"test.gte5Device1d": "pass",
"link.timeZone_TrueGeo": "pass",
"test.gte5CredentialDevice1d": "pass",
"test.credentialLTE500mi1hr": "pass",
"test.trueIPLTE500miInputIP": "pass",
"detect.vpn": "pass",
"test.exactIDAgeGTE7d": "fail",
"detect.proxyAnonymous": "pass",
"test.apSessionIDNotReplay": "pass",
"detect.jailbreak": "pass",
"blacklist.ip": "pass",
"test.expectedLanguage": "pass",
"link.proxyOrg_TrueOrg": "pass",
"detect.malware": "pass",
"detect.torExitNode90d": "pass",
"test.gte10Credential1d": "pass",
"detect.mobileTethering": "pass",
"test.trustedDevice28days": "fail",
"blacklist.telephone": "pass",
"test.gte20Credential1d": "pass",
"detect.aggregator": "pass",
"test.smartIDAgeGTE7d": "fail",
"detect.proxyOpenTransparent": "pass",
"detect.unusualActivity": "pass",
"detect.proxyHidden": "pass",
"test.credentialLTE50mi1hr": "pass",
"link.proxyISP_TrueISP": "pass",
"detect.tor": "pass",
"detect.torNode": "pass",
"test.telephoneAgeGTE30d": "pass",
"detect.knownVPNISP": "pass",
"link.proxyGeo_TrueGeo": "pass",
"test.gte2Credential1d": "pass"
}
}
],
"mbun": "xxxxx-xxxx-xxxx-xxxxx",
"forwardApiKey": "",
"policyObligation": false,
"policyDecision": "approve" // expect "approve", "deny"
}
Result
If policyDecision = approve
, the individual has been approved.
For instructions on how to test this workflow (including real user data), please visit: Testing MobileMatch Only
Updated 5 months ago